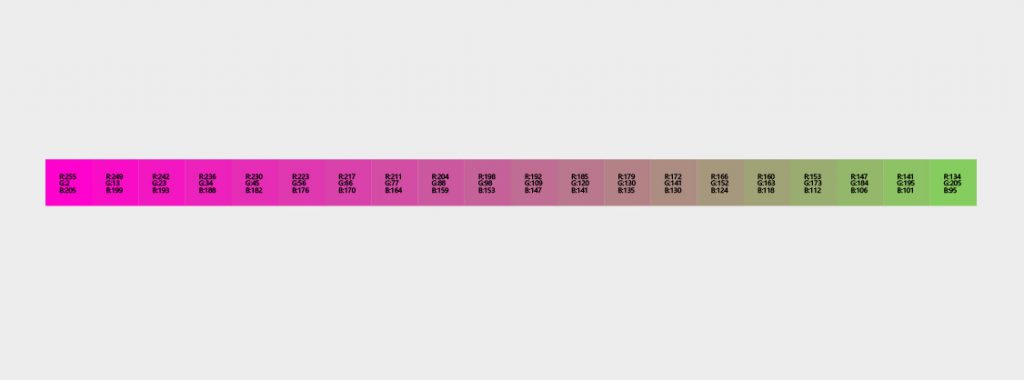
The basic functionality of this sketch is interrelating between to RGB values. Above is an array of 20 squares, each with a color that is interpolated between the two squares on each end. By decreasing the square or pixel size you can increase the fidelity of the gradient.
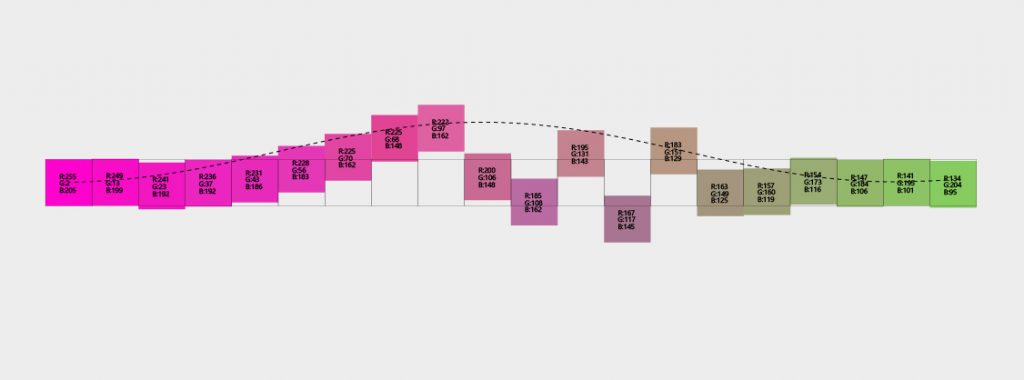
In this case we wanted the colors in the middle to diverge from the two colors the farther they are from the two ends (in this case the middle).
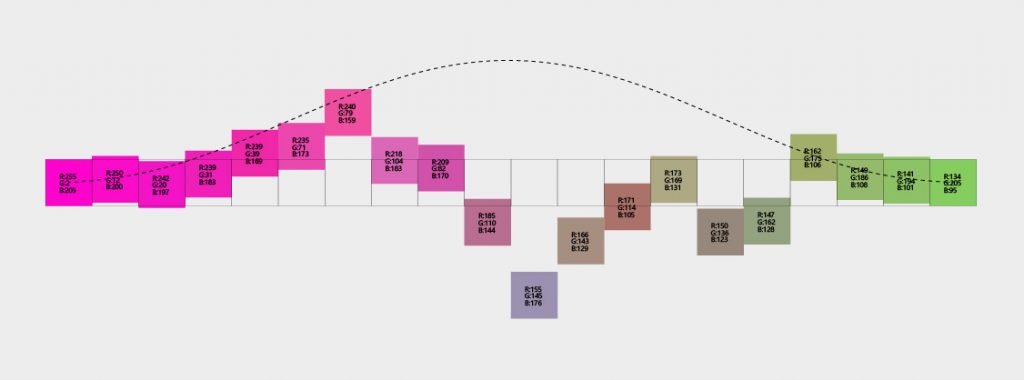
The distance from the ends can be multiplied to increase the domain of the random values that are added to the initial interpolated values.
We initially used this as a sketch for the initial concept for a hanging piece we designed for Behance’s new offices. Behance had just been acquired by Adobe and we wanted to create a piece that was made of the fundamental elements of their design suite: color and pixels. In this case, the piece is a three-dimensional form that hangs in the main stairway between he offices two fours, but the same principle applies: the initial gradient is driven by the distance between a point and then the color of each “pixel” diverges depending on its distance from that point.
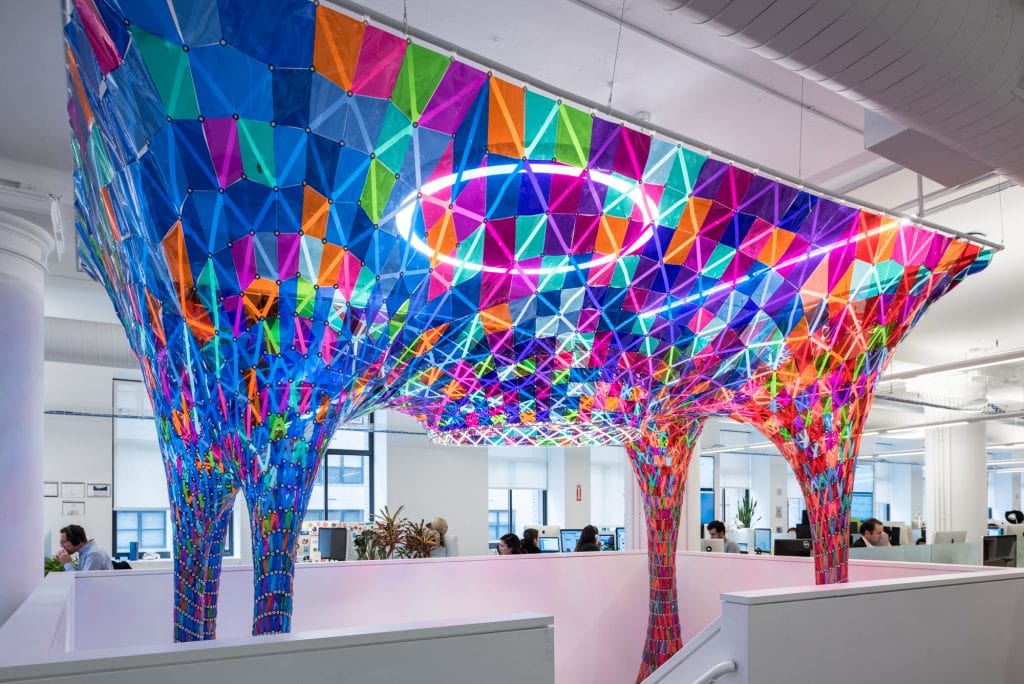
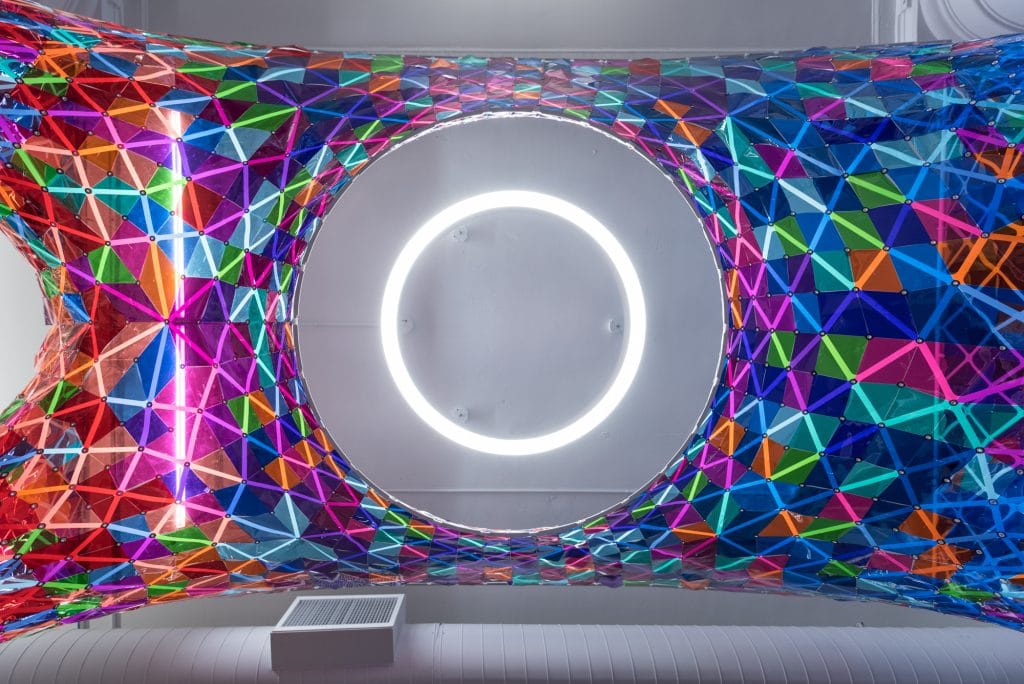
You can find the code for the initial processing sketch below:
import controlP5.*;
ControlP5 cp5;
int pixelsize;
int randomzone;
IntList R = new IntList();
IntList G = new IntList();
IntList B = new IntList();
int R1;
int G1;
int B1;
int R2;
int G2;
int B2;
int xstart = 100;
int ystart = 200;
int oldps;
int oldr;
int oldc;
boolean olda = true;
float r1;
float r2;
boolean stroke;
boolean additive;
void setup(){
size(displayWidth, displayHeight);
cp5 = new ControlP5(this);
cp5.addSlider("pixelsize")
.setPosition(100,20)
.setRange(5,200)
.setSize(240,20)
.setValue(10)
.setColorForeground(color(150,150,150))
.setColorLabel(color(255))
.setColorBackground(color(70,70,70))
.setColorValue(color(0,0,0))
.setColorActive(color(200,200,200))
;
cp5.addSlider("randomzone")
.setPosition(100,50)
.setRange(0,1000)
.setSize(240,20)
.setValue(255)
.setColorForeground(color(150,150,150))
.setColorLabel(color(255))
.setColorBackground(color(70,70,70))
.setColorValue(color(0,0,0))
.setColorActive(color(200,200,200))
;
cp5.addToggle("stroke")
.setPosition(100,80)
.setSize(20,20)
.setValue(true)
.setColorForeground(color(150,150,150))
.setColorLabel(color(255))
.setColorBackground(color(70,70,70))
.setColorValue(color(0,0,0))
.setColorActive(color(200,200,200))
;
cp5.addToggle("additive")
.setPosition(160,80)
.setSize(20,20)
.setValue(true)
.setColorForeground(color(150,150,150))
.setColorLabel(color(255))
.setColorBackground(color(70,70,70))
.setColorValue(color(0,0,0))
.setColorActive(color(200,200,200))
;
cp5.addSlider("R1")
.setPosition(450,20)
.setRange(0,255)
.setSize(240,20)
.setValue(0)
.setColorForeground(color(150,150,150))
.setColorLabel(color(255))
.setColorBackground(color(70,70,70))
.setColorValue(color(0,0,0))
.setColorActive(color(200,200,200))
;
cp5.addSlider("G1")
.setPosition(450,80)
.setRange(0,255)
.setSize(240,20)
.setValue(0)
.setColorForeground(color(150,150,150))
.setColorLabel(color(255))
.setColorBackground(color(70,70,70))
.setColorValue(color(0,0,0))
.setColorActive(color(200,200,200))
;
cp5.addSlider("B1")
.setPosition(450,50)
.setRange(0,255)
.setSize(240,20)
.setValue(255)
.setColorForeground(color(150,150,150))
.setColorLabel(color(255))
.setColorBackground(color(70,70,70))
.setColorValue(color(0,0,0))
.setColorActive(color(200,200,200))
;
cp5.addSlider("R2")
.setPosition(800,20)
.setRange(0,255)
.setSize(240,20)
.setValue(255)
.setColorForeground(color(150,150,150))
.setColorLabel(color(255))
.setColorBackground(color(70,70,70))
.setColorValue(color(0,0,0))
.setColorActive(color(200,200,200))
;
cp5.addSlider("G2")
.setPosition(800,80)
.setRange(0,255)
.setSize(240,20)
.setValue(0)
.setColorForeground(color(150,150,150))
.setColorLabel(color(255))
.setColorBackground(color(70,70,70))
.setColorValue(color(0,0,0))
.setColorActive(color(200,200,200))
;
cp5.addSlider("B2")
.setPosition(800,50)
.setRange(0,255)
.setSize(240,20)
.setValue(0)
.setColorForeground(color(150,150,150))
.setColorLabel(color(255))
.setColorBackground(color(70,70,70))
.setColorValue(color(0,0,0))
.setColorActive(color(200,200,200))
;
oldps = 0;
oldr = 0;
oldc = 0;
}
void draw(){
background(0);
int xsize = width-(xstart*2);
int ysize = height-(ystart*2);
int newc = R1+G1+B1+R2+G2+B2;
float psx = xsize/pixelsize;
float nsx = pixelsize + (xsize%pixelsize)/psx;
float psy = ysize/pixelsize;
float nsy = pixelsize + (ysize%pixelsize)/psy;
if((oldps != pixelsize) || (oldr != randomzone) || (oldc != newc) || (olda != additive)){
R = new IntList();
G = new IntList();
B = new IntList();
int i = 0;
for(int xc = 0; xc < psx; xc++){
for(int yc = 0; yc < psy; yc++){
float d = abs((xc*nsx+xstart) - (width/2));
float rth = 255 - (255 * d/randomzone);
if(rth < 0){
rth = 0;
}
if(additive == true){
r1 = 0;
r2 = rth;
}else{
r1 = rth * -1;
r2 = rth;
}
int radd = int(random(r1,r2));
R.append(int(((R2-R1)/(psx * 1.0) * xc) + R1)+radd);
radd = int(random(r1,r2));
G.append(int(((G2-G1)/(psx * 1.0) * xc) + G1)+radd);
radd = int(random(r1,r2));
B.append(int(((B2-B1)/(psx * 1.0) * xc) + B1)+radd);
i++;
}
}
}
int i = 0;
for(int xc = 0; xc < psx; xc++){
for(int yc = 0; yc < psy; yc++){
fill(R.get(i),G.get(i),B.get(i));
if(stroke == true){
stroke(1);
}else{
noStroke();
}
rect(xc*nsx+xstart,yc*nsy+ystart,nsx,nsy);
i++;
}
}
noStroke();
fill(R1,G1,B1);
rect(720,20,50,80);
fill(R2,G2,B2);
rect(1070,20,50,80);
oldps = pixelsize;
oldr = randomzone;
oldc = R1+G1+B1+R2+G2+B2;
olda = additive;
}