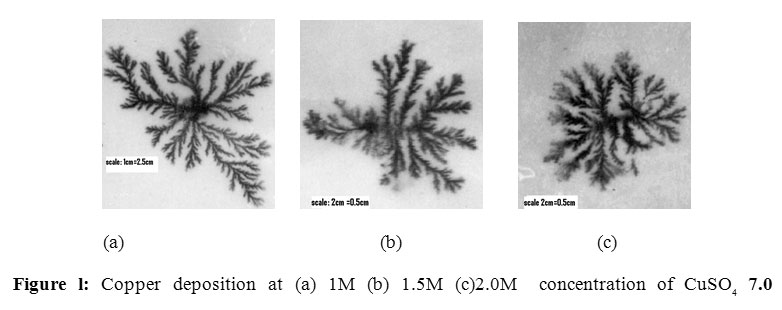
Diffusion-Limited Aggregation is an algorithm where particles aggregate together to form what appears to be a branching structure. Typically the particles move based on a Brownian motion or “random walker” algorithm. Particles aggregate when they are in proximity to a previously “dead” particle. As the aggregation grows it blocks off areas between aggregated particles creating a branching structure or Brownian tree. While DLA is often used to describe chemical reactions it is also visible everyday in other systems such as lightning, some types of choral and crystal growth.
The example above is a simplified version that does not use Brownian motion. Instead particles appear at a random position on circle and move towards the center until they reach an aggregated particle. The center particle starts with a random color and then passes that color with to the next aggregated particle with a random delta added to the color. This helps visualize the “genetic” history of each particle and change over time of the branches. The diagrams below show how the process works and you can find the Processing code for the sketch above.
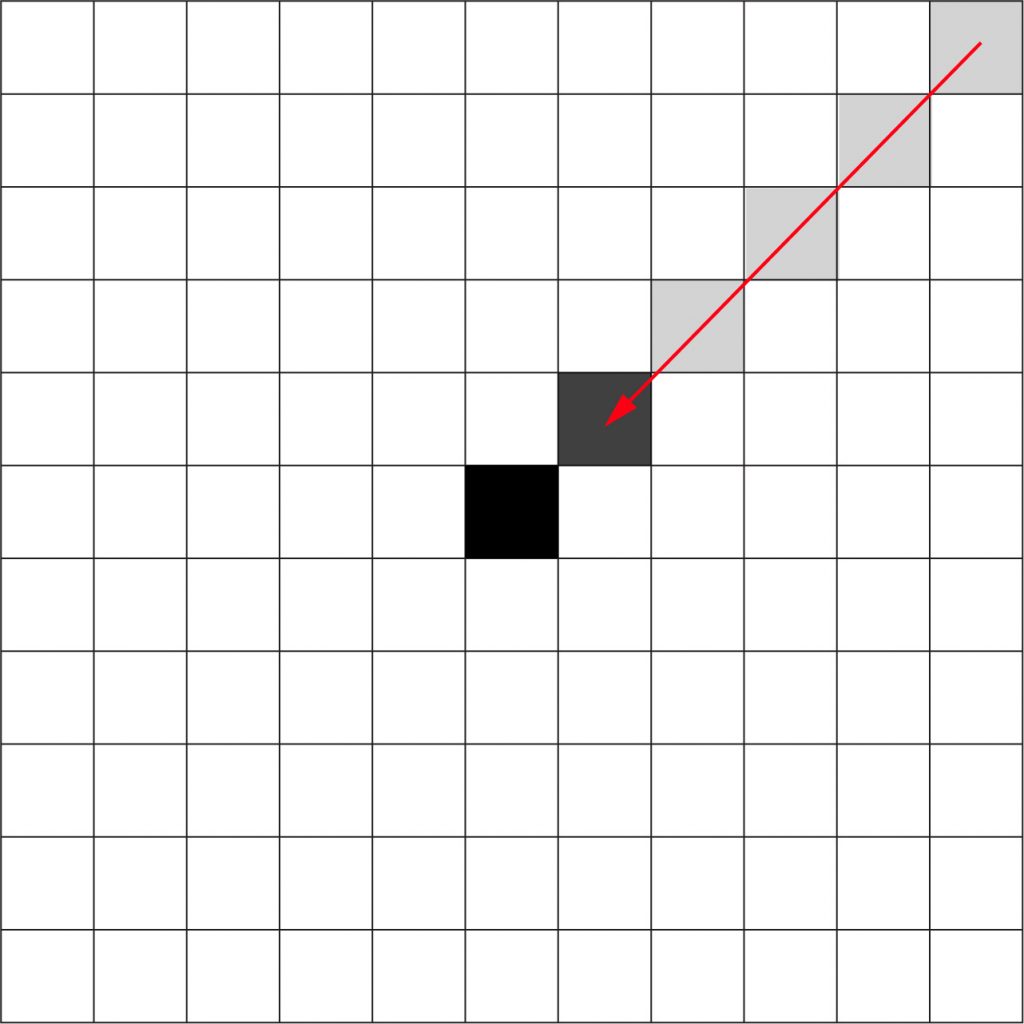
1. The center particle is static. All other particles move towards the center at a constant speed.
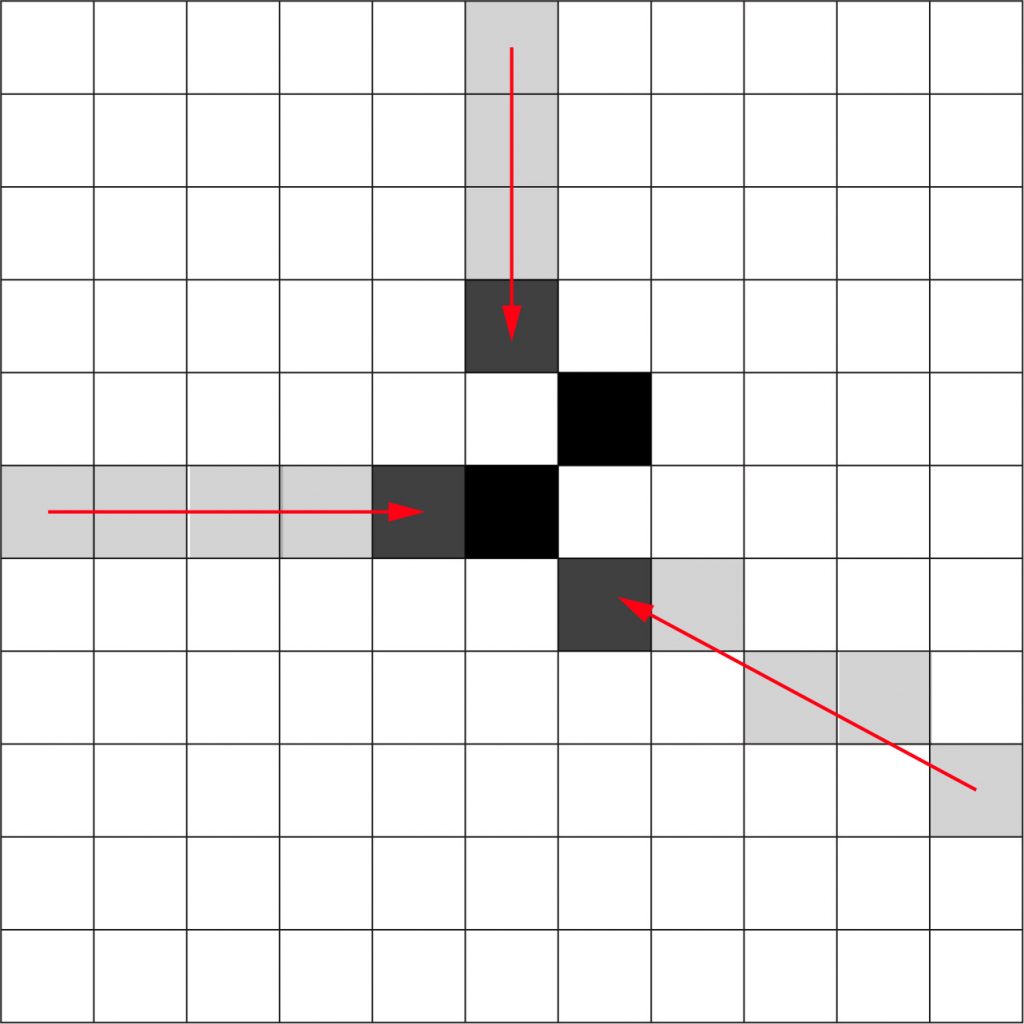
2. As a particle reaches a position adjacent to a static particle it becomes static
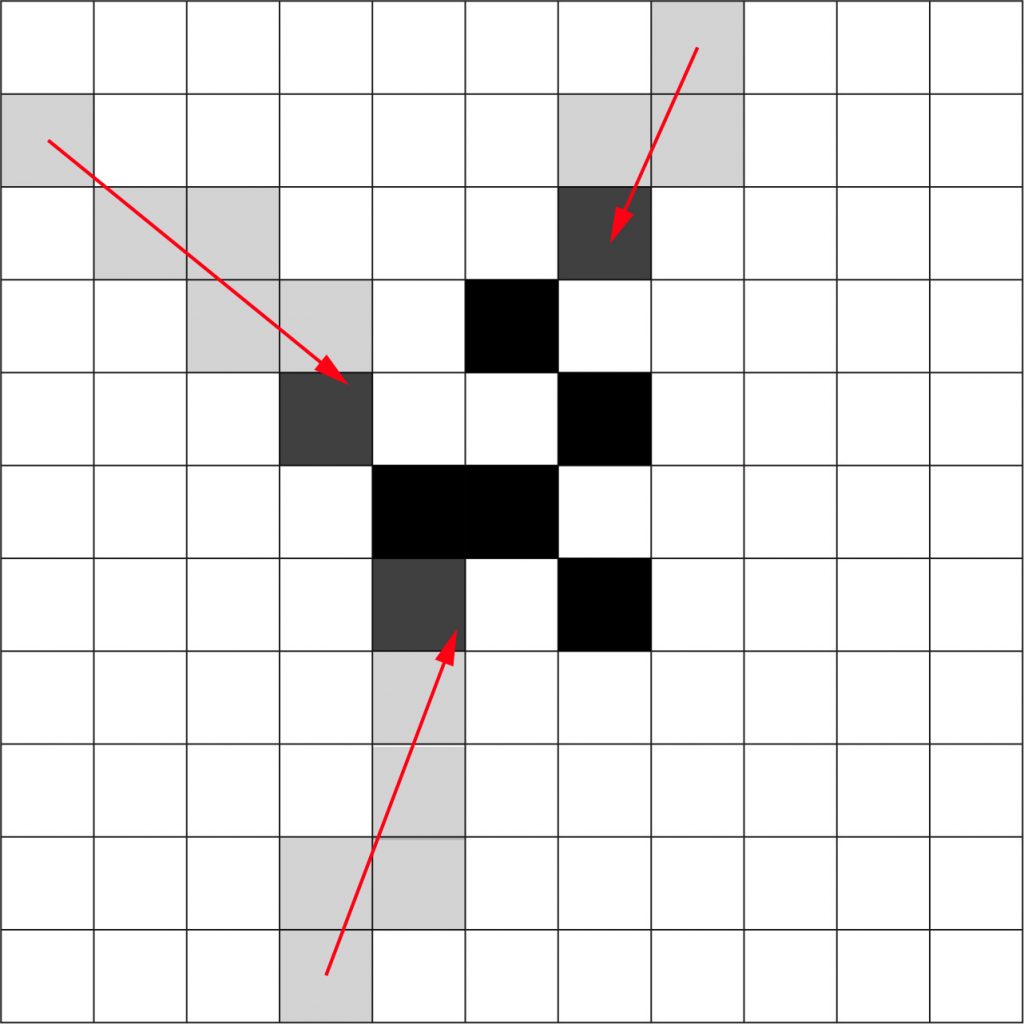
3. As particles become static they create an aggregated structure.
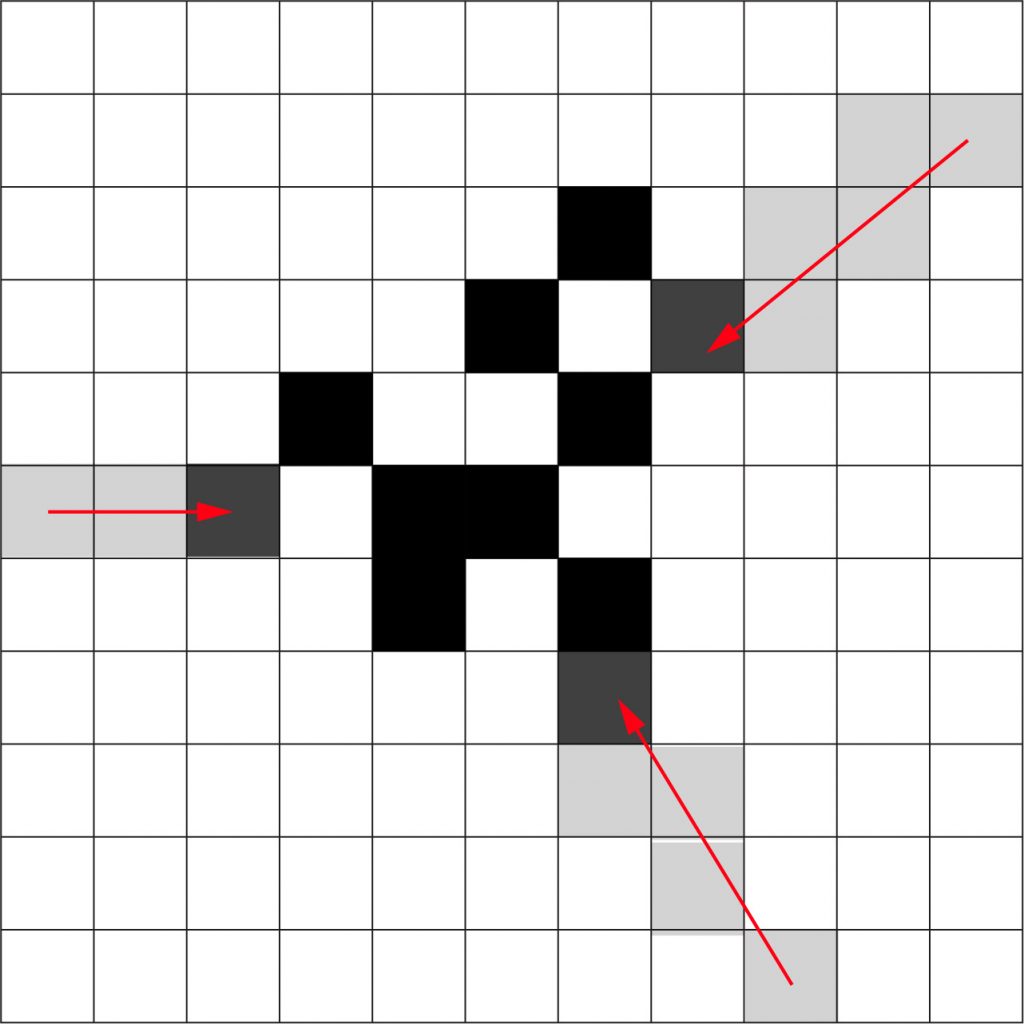
4. The aggregated structure branches as static particles block pockets of empty space.
//particle list
ArrayList <Particle> Particles = new ArrayList <Particle> ();
//define the distance between agregate (resolution)
int spacing = 3;
///particle count
int pc = 0;
//color range and speed increment
float crange = 30;
float spinc = 500;
void setup(){
size(900,900);
}
void draw(){
background(0);
//update particle list
for(int i = 0; i < Particles.size(); i++){
Particle pt = Particles.get(i);
pt.display();
pt.move();
pt.check();
}
//create new particle each frame
Particles.add(new Particle());
}
class Particle{
color cc;
float x,y,speedx,speedy;
int stop;
Particle(){
stop = 0;
cc = color(255,255,255);
//set random start posiiton on a cirlce
float ang = random(2* PI);
x = width/2 + (width/2.0) * cos(ang);
y = height/2 + (height/2.0) * sin(ang);
//define speed for particle
speedx = (width/2.0-x)/spinc;
speedy = (height/2.0-y)/spinc;
}
void display(){
stroke(cc);
strokeWeight(spacing);
point(x,y);
}
void move(){
if(stop == 0){
x = x + speedx;
y = y + speedy;
}
}
void check(){
//chceck to see if particle is first to reach center
if(dist(x,y,width/2,height/2) < spacing && pc < 1){
stop = 1;
x = width/2;
y = height/2;
cc = color(random(255),random(255),random(255));
pc++;
}
//check to see if particles position is within the spacing
//of an agregated particle and then set its color to a new color
//based on the agregated particles color and within the preset color range
for(int i = 0; i < Particles.size(); i++){
Particle pt = Particles.get(i);
if(dist(x,y,pt.x,pt.y) < spacing && pt.stop == 1 && stop == 0){
stop = 1;
float R = red(pt.cc) + random(-crange,crange);
float G = green(pt.cc) + random(-crange,crange);
float B = blue(pt.cc) + random(-crange,crange);
cc = color(R,G,B);
pc++;
}
}
}
}